(c) Scott M. Baker, smbaker@primenet.com
Purpose:
C# Slot Machine in 15minutes: Here i teach you how to make a basic slot machine in 7 minutes, with a further 8 minutes of explanation! Help with C# coding. Slot Machine Simulation: A slot machine is a gambling deviceinto which the user inserts money and then pulls a lever (orpresses a button). The slot machine then displays a set of randomimages.
SBSlot is a slot machine simulator. There are presently two separate programs included in this package:
- SlotStat.Exe: Statistical slot simulator. Used for obtaining an accurate simulation of the odds/payoffs of a slot machine. Text-based output format. Capable of automatically simulating the slot machine for millions (billions?) of pulls.
- Slot3D.Exe: Graphical 3D slot game. This requires Microsoft's DirectX 3.0 package for Windows-95. This program is intended to function as a realistic slot machine game. If you need DirectX3, try searching my directX links page at http://smbaker.simplenet.com/directx.html.
Installation:
You can run the automated install program (Setup.Exe) or you can copy the files manually into the directory of your choice. SlotStat.Exe is the statistical program and Slot3d.Exe is the graphical game.
SlotStat Operation:
The initial screen will ask you to load a file; A few are supplied for you to use. Once the slot machine file has been loaded, the screen should display the initial state of the machine (i.e. no credits, reels at initial positions, etc).
Press 'Pull' to pull the handle one time.
Press 'Run' to initiate automatic mode; The machine will be pulled several times per second, simulating game play.
Press 'Stop' to stop automatic mode.
Press 'Reset' to reset the statistical data at any time.
There is also a checkbox marked '100x Speed Mult'. This will make the machine operate 100 times faster than the normal speed.
Slot3D Operation:
The initial screen will ask you to load a file.Once loaded, you should get a 3D window displaying the slot machine. You can operate the machine by pressing the yellow buttons or by using the pull-down menu options.
You can pan the screen up/down/left/right by using the scrollbar controls on the window borders. You can zoom in and out using the +/- keys or the Display menu option.
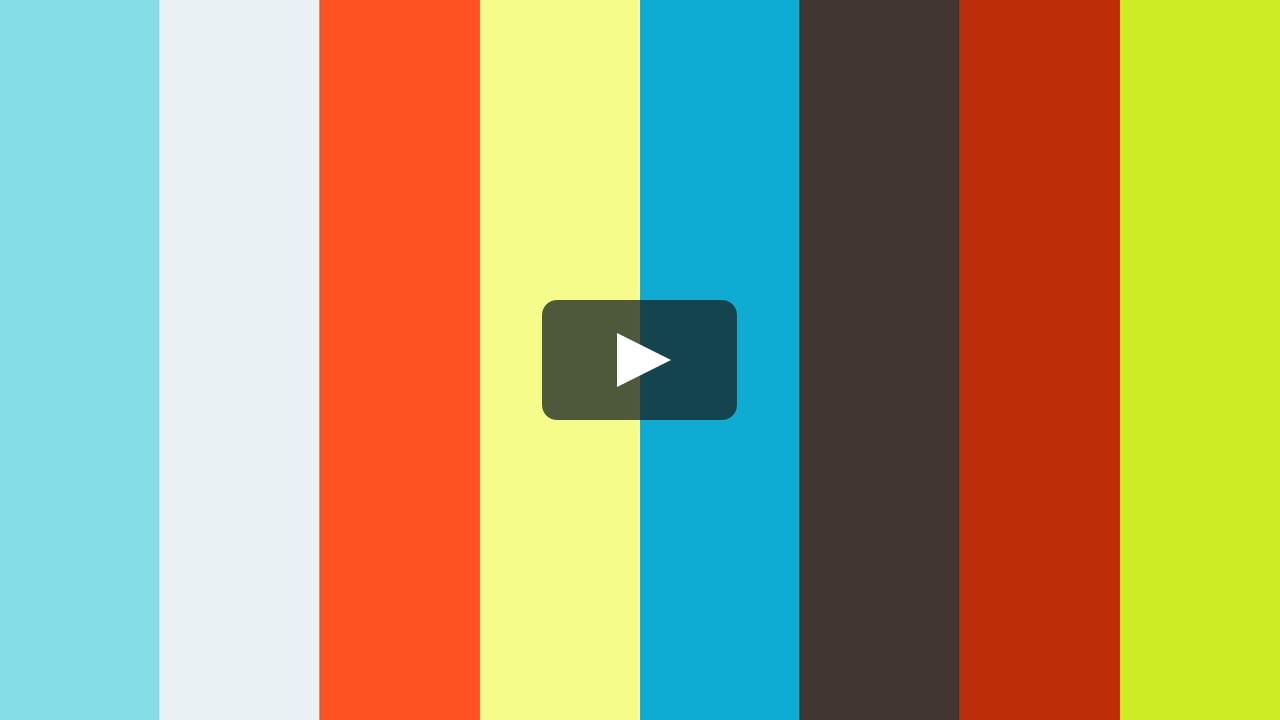
Several yellow buttons are present on the slot machine:
- [Change]: Cashes in some boney for credits. It'll automatically repeat the last cashing that you did. Defaults to $20. You may select other denominations using the pull-down menu.
- [Cash/Credit]: In a real machine, this button cashes out your money. Since your PC doesn't have a Coin Hopper attached to it, this button doesn't do anything.
- [Bet 1]: If you want to bet less than the 3-credit maximum, then you can bet one credit at a time.
- [Spin]: Assuming you have used [Bet 1] to bet one or more credits, you can spin the reels.
- [Play 3]: This plays three credits and spins the reels automatically.
There are several display options located under the Render and Display menus. These modify the 3D rendering options and control a few other visual aspects.
Note: If you are getting a very low FPS (Frames Per Second) rate, you might want to disable (uncheck) the 'Display Payoff Chart' option.
Slot Machine Basics:
Historically, there have been several different types of slot machines:
- Early Mechanical. The really old 'antique' slots.
- Electro-Mechanical. Solenoids and relays added to give more complex game capabilities and improve reliability. Reels are still mechanical, but the electronic parts perform functions like multiplication, detecting payline symbols, accepting multiple coins, running lights & sounds, etc.
- Video. Rather than using mechanical reels, reels are displayed as 'graphics' on a video screen. Probabilities/Odds entirely determined by computer.
- Modern Electronic. Computerized, but with stepper motor reels. Made to look and feel like a mechanical machine, but it's really all computer based.
With the earlier machines (Mechanical and Electro-Mechanical), the probabilities are entirely determined by the symbols on the reels. You can simply read the symbols off the reels and input the data into SBSlot for a simulation.
The newer machines (Video and Modern Electronic) are a bit more difficult. These machines typically have some sort of virtual reels encoded into the computer. These encodings may or may not be representative of what you see in their displays.
Most video machines can activate a 'reel test' diagnostic which will display the contents of the 'virtual reels' to the video screen. These virtual reels can be quite large, up to even 256 symbols per reel. Although it takes a long time to get all these symbols copied down, the layout is relatively straightforward.
Modern Electronic machines (such as IGT's s+ series) also have a reel test mode. However, since these machines do not have a video display, it can't simply dump the information out to you. Instead, the machines must display the contents of their 'virtual reels' on the 'physical reels'. This amounts to having to spin their 'physical reels' to display each symbol on the 'virtual reel'.
An important note here is that the physical reel is NOT the same as the virtual reel in the slot machine's computer. You cannot take the symbol information off the physical reels! What the slot manufacturers have done amounts to fiddling around with the probabilities a bit.
Note that one machine isn't the same as another. The chips on the motherboard determine the contents of the 'virtual reel'. Thus, one double diamond machine may have a very different payoff from another.
Slot Machines Included
bertha.slt: Bally 'big bertha' $1 video slot. This is a mammoth machine, standing about six feet tall. It's got a big 27' video screen. The internal configuration of the machine is such that there are 3 reels each with 255 symbols per reel.
golden.slt: This is another Bally video slot. This one has four reels, each with 64 symbols per reel. This is a $5 token machine, and has a payoff of $5,000,000 if you hit four golden nuggets straight across. Of course, there's only 1 golden nugget on each reel, leading to a probability of 1/64^4.
doubled.slt: This is an IGT double diamond deluxe, one of the state-of-the-art machines you can currently find in casinos. It's a modern electronic machine. The physical reels have 11 symbols and 11 blank spots each, but the 'virtual' reels have 72 symbols each,.
The game has a few special features, including symbols that 'clunk down' if they appear above the payline. Thus, while there are a large number of blank spaces on the reels, many of them are adjacent to a bar which will clunk down and award you a jackpot. Note: SlotStat doesn't currently display the 'clunk' itself, but it does account for it and display the final results correctly.
Note: The machines and trademarks mentioned above are copyrighted by their respective manufacteres, Bally, and International Gaming Technology (IGT).
.slt files
Slot machine data is stored in .slt files. The format is as follows:
There are three sections, for symbols, reels, and payoffs respectively. Each section is seperated by a '%%'. The final section should have a '%%' after it.
You'll also find several special tags that are specified by a percent sign and a name, for example '%sndmusic'. These are used to specify various attributes. Most should be fairly self-evident if you want to tinker around with them.
symbols:
The format is:
[character] [filename] [description]
Fields are specified as follows:
- [character]: One-letter identifier that you'll use to refer to the symbol in the reel and payoff sections.
- [Filename]: Identifies the image that will be displayed in the Graphical 3D version. All files are assumed to be BMP files and no extention is specified. Direct3D handles textures better if multiple textures are grouped into the same file (i.e. four textures stored in a 256x256 file is better than four 64x64 files). There are several ways to specify the texture filename/format:
- Simple filename (no extention). The entire file will be treated as the texture for the symbol.
- Filename (no extention) with a slash and a number [1-4]. The file will be treated as a 2x2 grid of textures. The numbering works as follows:
filename/1 filename/2 filename/3 filename/4 - Filename (no extention) with a slash and a letter [a-p]. The file will be treated as a 4x4 grid of textures. (sym256.bmp and sym512.bmp are set up this way) The numbering works as follows:
filename/a filename/b filename/c filename/d filename/e filename/f filename/g filename/h filename/i filename/j filename/k filename/l filename/m filename/n filename/o filename/p - The single string 'blank'. This will be treated as a pure white (RGB=1,1,1) ;space, and not read from an actual bmp file.
- [description]: Textual description/name of the symbol.
You can also prefix a symbol by (wild) if it is wild, (double) if it should double the jackpot, (up) if it should 'clunk' up, and (down) if it should clunk down.
'*' is a special symbol meaning 'any' and should not be used here.
Example, 'a 1bar single bar'
reels:
All reels are speficied on one line, with one reel symbol per line. (That probably didn't make any sense. here's an example)
[reel 1 sym 1] [reel 2 sym 1] [reel 3 sym 1]
[reel 1 sym 2] [reel 2 sym 2] [reel 3 sym 2]
...
[reel 1 sym n] [reel 2 sym n] [reel 3 sym n]
I hope that made more sense. Here's a concrete example:
c 1 2
7 3 3
g 0 g
Reel #1 would have the symbols 'c', '7', and 'g' (top to bottom). Reel #2 would have '1', '3', and '0'. Reel #3 would have '2', '3', and 'g'.
Payoffs:
This is the most complicated section. Each payoff begins with a character for each reel symbol. Then theres a carat (^) followed by one or more payoff amount (in coins), followed by another carat (^) followed by a textual description.
For example,
'c c c ^ 2 ^ Cherry-Cherry-Cherry', This could mean 2 credit payoff for three cherries
You can use a + to specify the symbol can be above or below the payline as well. For example,
Slot Machine Simulation C#
'+g +g +g +g ^ 1000 ^ gold-gold-gold-gold', This could mean four golden nuggest left to right, on above or below the payline.
Enclosing multiple symbols in braces means any symbol could match. For example,
'[123] [123] [123] ^ 100 any bar-any bar-any bar', could be used to match any series of bars. (assuming 1 = single bar, 2 = double bar, 3 = triple bar)
You can use an asterisk (*) to denote anything (including a blank) may match. For example,
'c * * ^ 1 ^ cherry-any-any', could be used to match a single cherry.
Revision History
- Version 1.0
- Initial Public Release
- Version 1.1
- Fixed problem with each 'pull' being counted twice
- Fixed payoff problems in golden nugget
- Version 1.2
- Finsihed 3D version.
Contacting me:
You can reach me by email at smbaker@primenet.com.
You can find sbslot at
Slot Machine Simulator C# Download
http://smbaker.simplenet.com/sbslot/sbslot.html.
--- or ---
http://www.primenet.com/~smbaker/sbslot/sbslot.html.
- Appendices
- Slots Analysis
- Miscellaneous
Introduction
When it comes to gambling, the easier a game is to understand the worse the odds usually are. This is certainly the case with slot machines. Playing them is as easy as pressing a button. However, between the high house edge and fast rate of play, there is no quicker way to lose your money in a casino.
Before going further, let me make clear that this page addresses the way slot machines work in most parts of the United States and the world. However, some parts of this page do not apply everywhere. For example, I state that slot machines have a memory-less property, where the odds of every spin are the same. In some places, like the UK, some machines in bars, called 'fruit machines,' have a mechanism that guarantees a certain profit over the short run, which causes the game to go through loose and tight cycles. These games do not have the usual independence property of the major slot makers.
How They Work
Whether you're playing a 3-reel single-line game or a 5-reel 25-line game, the outcome of every bet is ultimately determined by random numbers. The game will choose one random number for each reel, map that number onto a position on the reel, stop the reel in the appointed place, and score whatever the outcome is. In other words, the outcome is predestined the moment you press the button; the rest is just for show. There are no hot and cold cycles; your odds are the same for every spin on a given machine.
Slot machines are just about the only game in the casino where the odds are not quantifiable. In other words, the player doesn't know how the game was designed, so it is difficult to look at an actual game to use as an example. So, to help explain how they work, I created the Atkins Diet slot machine (link). It is a simple, five-reel game with a free spin bonus round, much like IGT's Cleopatra game.
For information on how it works and all the odds, please visit my Atkins Diet par sheet.
For a more complicated example, featuring sticky wilds in the bonus, please try my Vamos a Las Vegas slot machine.
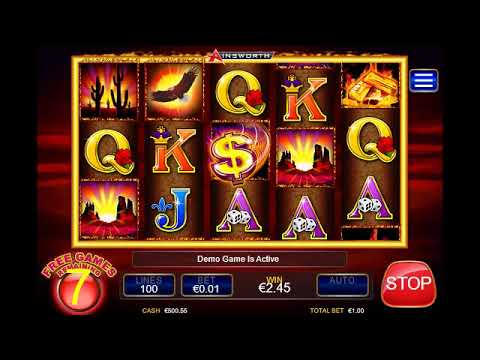
For information on how it works and all the odds, please visit my Vamos a Las Vegas par sheet (PDF).
Odds
The following table shows the casino win for Clark County Nevada (where Las Vegas is) for all slots for calendar year 2012. They define 'slot' as any electronic game, including video poker and video keno. I've found video keno to be about equally as tight as reeled slots, but video poker has a much higher return. So, the return for reeled slots should be higher than these figures.
Clark County Slot Win 2012
Denomination | Casino Win (pct) |
---|---|
$0.01 | 10.77% |
$0.05 | 5.96% |
$0.25 | 5.74% |
$1.00 | 5.64% |
$5.00 | 5.51% |
$25.00 | 3.97% |
$100.00 | 4.73% |
Megabucks | 12.89% |
Multi-denomination | 5.32% |
Total | 6.58% |
Source: Nevada Gaming Control Board, Gaming Revenue Report for December 2012 (PDF, see page 6).
Most players play penny video slots. Based on past research, I find the house edge on those to usually be set from 6% to 15%. In general, the nicer the casino, the tighter the slots.
Advice
While there is no skill to playing slots, there is some skill in selecting which machine to play and ways you can maximize your return. What follows is my advice, if you must play slots at all.
- Always use a player card. Slots may be a lousy bet, but the casinos treat slot players very well. A $1 slot player will probably get comped better than a $100 blackjack player. Of course, don't play for the reason of getting comps. You'll give them a lot more than they'll give you.
- The simpler the game, the better the odds. The fancy games with big signs and video screens tend to not pay as well as the simple games. However, slot players always tell me the fancy games are more fun.
- The higher the denomination, the better the odds. For that reason, it is better to play one coin per line on a 5-cent game than five coins per line on a 1-cent game.
- Don't forget to cash out and take your ticket when you leave. It is easy to forget after hitting a jackpot.
- Try to play slowly and as little as possible to get your fix.
- In some games there is a skill feature, like Top Dollar. In such games, advice is usually offered, which you should take.
Myths and Facts
Just about everything that players believe about slots is untrue. Here are the most common myths and facts. As a reminder, this page is based on slot machines commonly found in the United States. Some machines, like 'fruit machines' found in the United Kingdom work differently.
- Myth: Slot machines are programmed to go through a cycle of payoffs. Although the cycle can span thousands of spins, once it reaches the end the outcomes will repeat themselves in exactly the same order as the last cycle.
Fact: This is not true at all. Every spin is random and independent of all past spins.
- Myth: Slot machines are programmed to pay off a particular percentage of money bet. Thus, after a jackpot is hit the machine will tighten up to get back in balance. On the other hand, when a jackpot has not been hit for a long time it is overdue and more likely to hit.
Fact: As just mentioned, each spin is independent of all past spins. That means that for a given machine game, the odds are always the same. It makes no difference when the last jackpot was hit or how much the game paid out in the last hour, day, week, or any period of time.
- Myth: Machines pay more if a player card is not used.
Fact: The mechanism that determines the outcome of each play does not consider whether a card is used or not. The odds are the same with or without one.
- Myth: Using a player card enables the casino to report my winnings to the IRS.
Fact: That makes no difference. If you win $1,200 or more they will report it either way. If you have a net losing year, which you probably will, at least the casino will have evidence of it. Such annual win/loss statements may be used as evidence to declare offsetting loses to jackpot wins.
- Myth: The slot department can tighten my game with the press of a button remotely. Thus, you better be nice to the staff and tip them well, or they will use a remote control to have the machine take you down in a hurry.
Fact: There is now some truth to the myth that the odds of a machine can be changed remotely. Such 'server-based slots' are still experimental and in a minority. Even with server-based slots, there are regulations in place to protect the player from the perceived abuses that could accompany them. For example, in Nevada a machine can not be altered remotely unless it has been idle for at least four minutes. Even then, the game will display a notice that it is being serviced during such changes. (source) Meanwhile, for the vast majority of slots, somebody would physically need to open the machine and change a computer chip, known as an EPROM chip, to make any changes.
- Myth: The machines by the doors and heavy traffic flow areas tend to be loose while those hidden in quiet corners tend to be tight.
Fact: I've studied the relationship between slot placement and return and found no correlation. Every slot director I've asked about this laughs it off as just another player myth.
- Myth: Slots tend to be looser during slow hours on slow days of the week. However, when the casino is busy they tighten them up.
Fact: Nobody would take the trouble to do this, even if he could. The fact of the matter is the casinos are trying to find a good balance between winning some money while letting the player leave happy. That is best achieved by slots loose enough to give the player a sufficiently long 'time on device,' as they call it in the industry, with a reasonable chance of winning so he will return to the same casino next time. If the slots are too tight, the players will sense it and be unlikely to return.
The kind of place you're likely to find tight slots are those with a captive audience, like the Las Vegas airport. So, if the slot manager feels that 92% is the right return for a penny game, for example, he is likely to set every penny game all that way, and keep them that way for years.
Play
Analysis
Vamos a Las Vegas
Analysis (PDF). Australian Reels — One Line
Analysis (PDF)
Australian Reels — Five Line
Analysis
21 Bell
Analysis
Fruit Machine
Analysis
Slot Machine Simulator C# Simulator
Reviews
- Dazzle Me (NetEnt)
- Mr. Vegas (Betsoft)
- Sparks (NetEnt)
Internal Links
Slot Machine Simulator C# Demo
- Appendix 1 shows the details and analysis of almost 4000 actual spins on a Reno slot machine.
- Appendix 2 shows an example of the virtual reels behind a hypothetical slot machine and how the average return is calculated.
- Appendix 3A: 2003 Las Vegas slot machine rankings.
- Appendix 3B: 2002 Jean/Primm slot machine rankings.
- Appendix 3C: 2002 Tunica slot machine rankings.
- Appendix 3D: 2002 Henderson/Lake Mead slot machine rankings.
- Appendix 3E: 2002 Quarter and dollar returns for Las Vegas slots
- Appendix 4 shows how the return is calculated for my Wizard's Fruit Slot Machine.
- Appendix 5 analysis of the 21 Bell Slot Machine.
- Appendix 6 Analysis of Red, White, & Blue Slot Machine.
- Lock and Roll analysis of the skill-based slot machine found in North Carolina.
- Deconstructing Jackpot Party analysis of the video slot machine.
- Deconstructing Lion's Share analysis of the classic MGM progressive game.
- Deconstructing Cleopatra analysis of the popular IGT game.
- Deconstructing Lionfish analysis of the slot game found on many Game Maker machines.
- Deconstructing Megabucks.
- Deconstructing the Atkins Diet slot machine.
- Deconstructing Lucky Larry's Lobstermania.
- Deconstructing Hexbreaker.
- Deconstructing Blazing Sevens.
- Deconstructing Hot Roll.
- Mystery progressives on Ainsworth slots.
- Mystery progressives on WMS slots.
- Baltimore Sun article, in which I am quoted.
- 100% Rebate on Slot Losses Promotions: When to quit playing when all losses are refunded.
External Links
- For a simplified explanation of slots, please see my companion site Wizard of Vegas
- German translation of this page is available at richtigspielen.com
- Another decent overview of how slots work and some practical advice for playing them is How Slot Machines Work at VegasClick.com.
- PAR Sheets, probabilities, and slot machine play: Implications for problem and non-problem gambling by Kevin A. Harrigan and Mike Dixon, University of Waterloo, Waterloo, Ontario, Canada. This is an outstanding academic paper that details how some popular slot machines were designed.
- PAR Sheets, probabilities, and slot machine play: Implications for problem and non-problem gambling - Academic paper based on the par sheets for some modern slot machines
Written by: Michael Shackleford